Using DLLs in Automation 360
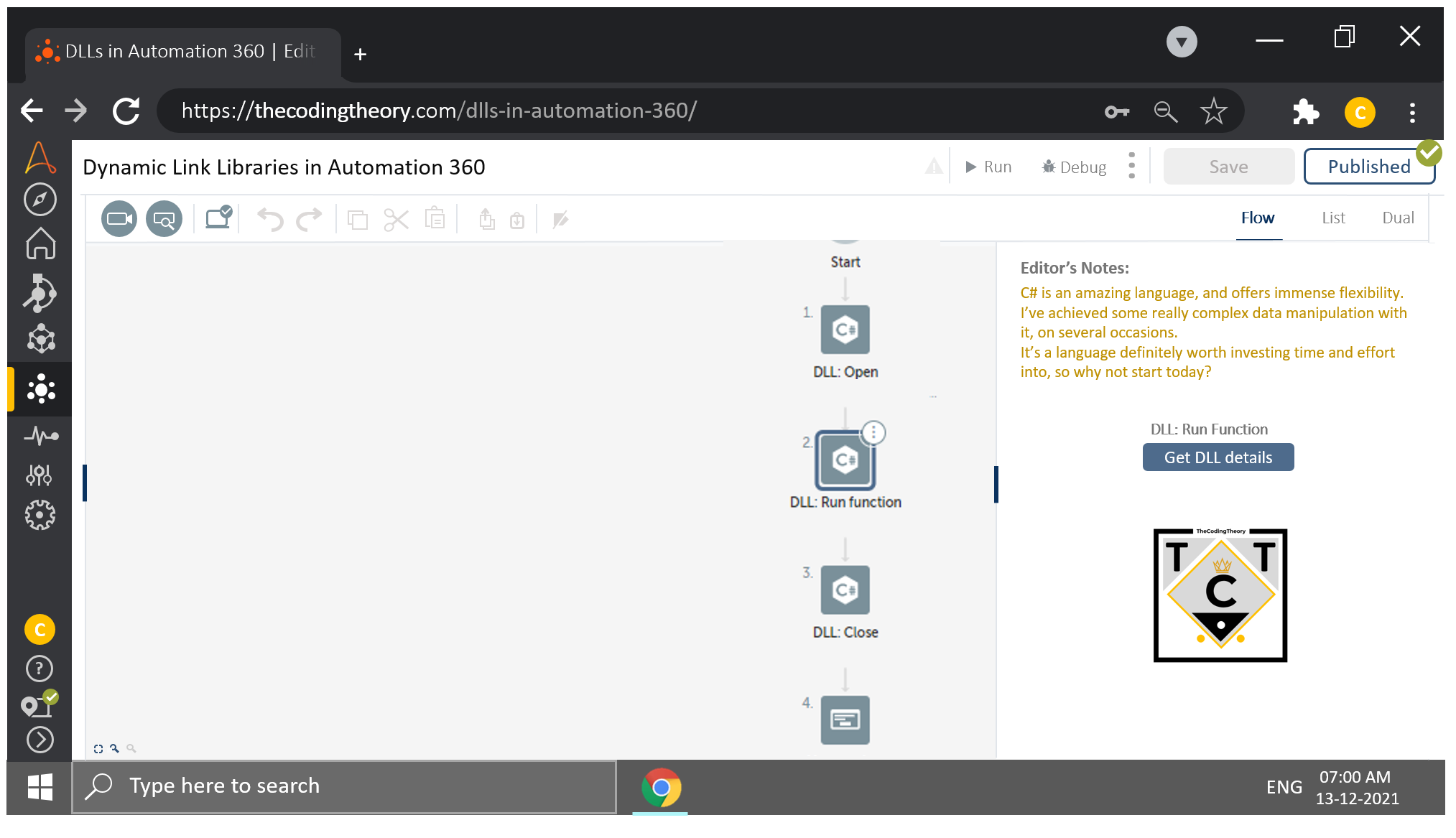
C# is a fairly advanced programming language.
But don’t let that “fairly advanced” scare you off.
Like most programming language, C# is easy if you study the basic concepts that govern it. If you nail a concept or two each day, then learning C# will be a walk in the park…
Provided you have a truckload of nails, because that’s the number of concepts you’ll have to hammer down before you can even think of developing something worthwhile.
But don’t let that “truckload of nails” scare you off.
There is nothing fairly advanced about nailing down concepts.
I Don’t Want to Learn It Anymore.
If you’ve made it this far then that means you can tolerate me, and if you can do that then learning C# will be a cakewalk.
I mentioned earlier that C# is a fairly advanced programming language, and by that I meant it uses objects to get things done. These objects allow us to represent complex items very easily, which is why languages that use objects are so popular.
I bet that flew right over your head, but no matter.
Everything seems scary at first, but that won’t stop you from becoming a full stack dabble-oper like yours truly.
Dabble me This!
It is often assumed that to stay afloat in the oceans of IT, one must explore new territories, or dive to depths most wouldn’t ever dream of.
While this is true for the most part there is another way – and that is the way of the Dabbler.
Simply put, you don’t have to understand everything in depth – strive to understand whatever you know well enough to implement practical solutions.
Love designing PowerPoint illustrations?
Stick with creating illustrations long enough, and you will eventually become a pro and might even come up with your own unique art style.
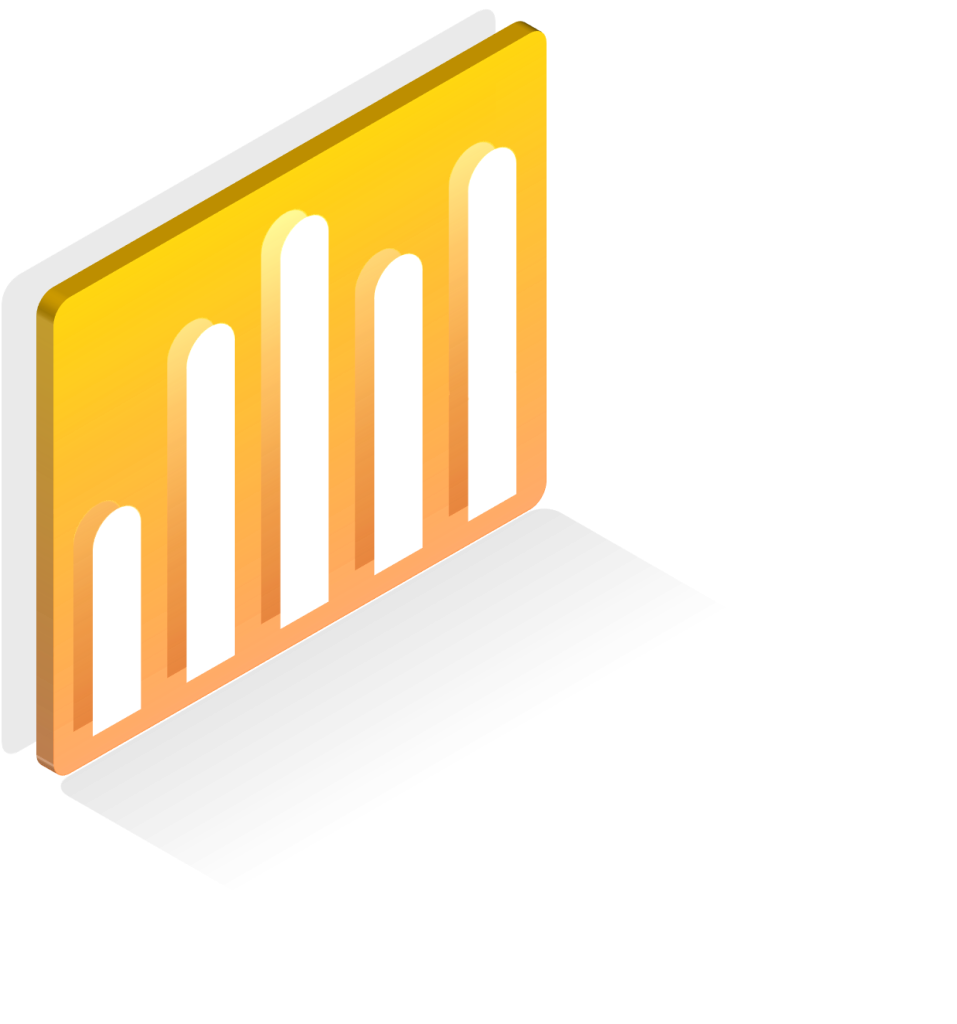
Love tinkering around with Excel and Macros?
Keep at it, and you will eventually reach a level of expertise which will enable you to freelance, or even start a YouTube channel teaching others using a Tool most IT “professionals” would like to think are “old-school” or “deprecated”, or even “worthless”.
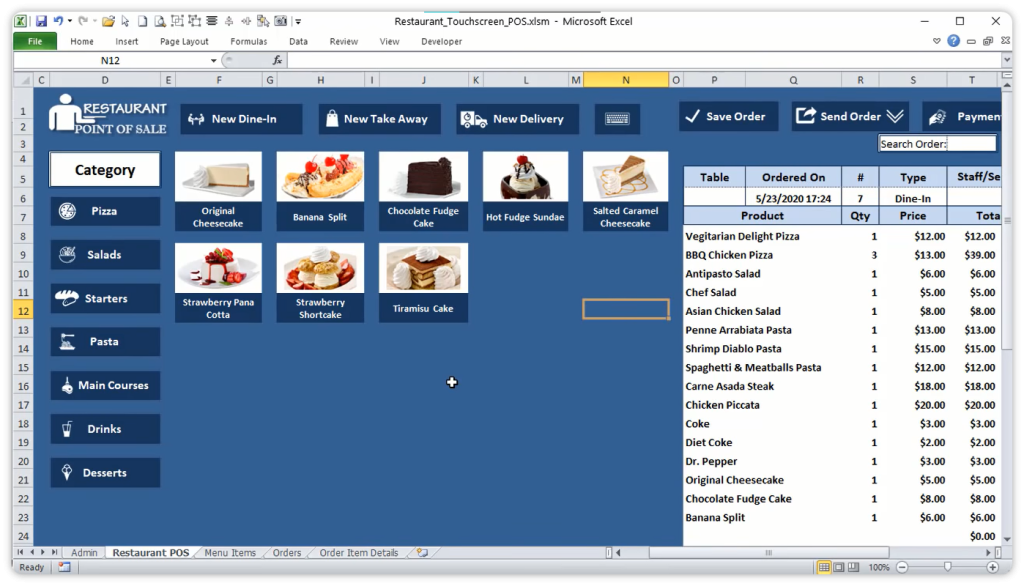
Try saying that to the Microsoft MVP and Founder of Excel for Freelancers.
Love music so much that you want to become a musician one day?
Your parents are going to be very disappointed.
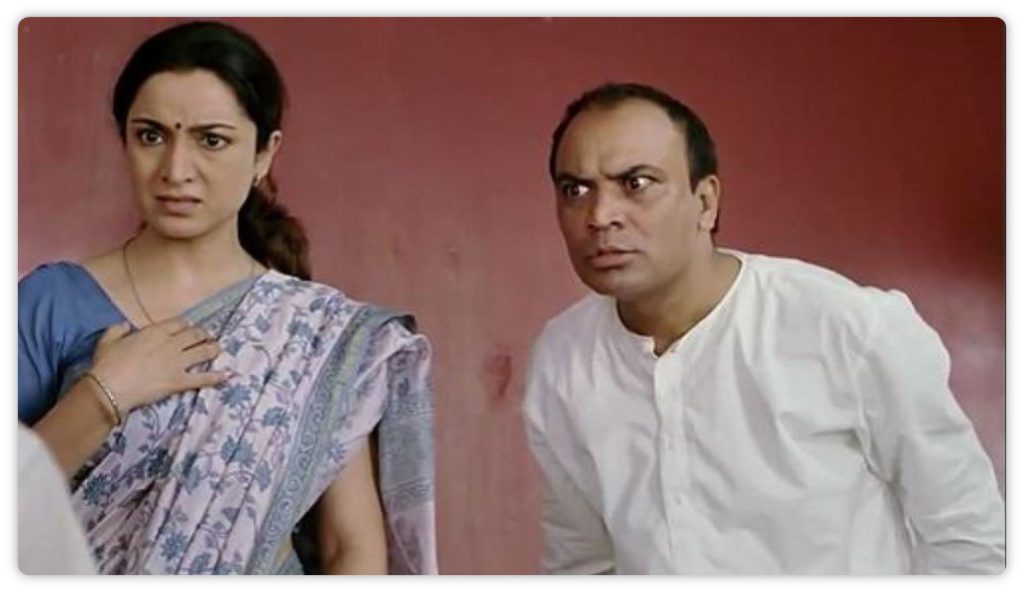
Then again, all of this is easier said than done.
How would we go about achieving this mystical balance between getting lost in the mountains and getting eaten up by sharks?
By becoming a Jack.
Don’t Pick a Trade, Pick Em’ All!
I am not a C# developer, but I have dabbled in a couple of concepts which helped me understand it well enough to leverage it to my advantage. The point here is to expose yourself to as many concepts as you possibly can BEFORE settling on a tool, because when you decide on the tool, you will also have an idea as to which all scripting languages will enhance your experience and flexibility with that particular tool, making your life as a software developer easier…ish.
C# just happens to be one of these languages that I personally think is an amazing fit. Python, VBScript and JavaScript can be quite useful as well – its up to you.
Some prefer working primarily with Python, while some don’t. It all boils down to a matter of preference, but if you don’t expose yourself to them, you won’t even consider learning them in the first place, now would you?
Let’s expose ourselves to one such example, just to show you how C# can be useful when integrated with RPA.
On the side, I am also preparing a series of exercises and walkthroughs on this very topic, but I’ve been stuck “preparing” for quite some time now.
I’ve run out of excuses to make at this point.
Converting String to a Desired Date Format
Yes, yes, we have a DateTime Action Package for that, but let’s start with something simple instead of diving into complex scenarios.
Over to Visual Studio.
Now remember, Automation Anywhere only accepts Dynamic Link Libraries, so you can’t create a Console Application and expect it to operate with that.
You have to develop methods inside something called a Class Library.
As the name suggests, it’s a Library of Methods. You can browse through the catalogue of Methods, pick the class you want from the shelf, flip through the Methods and bookmark the ones you want.
Really bad analogy, but you get the idea.
Lets create a New Project first.
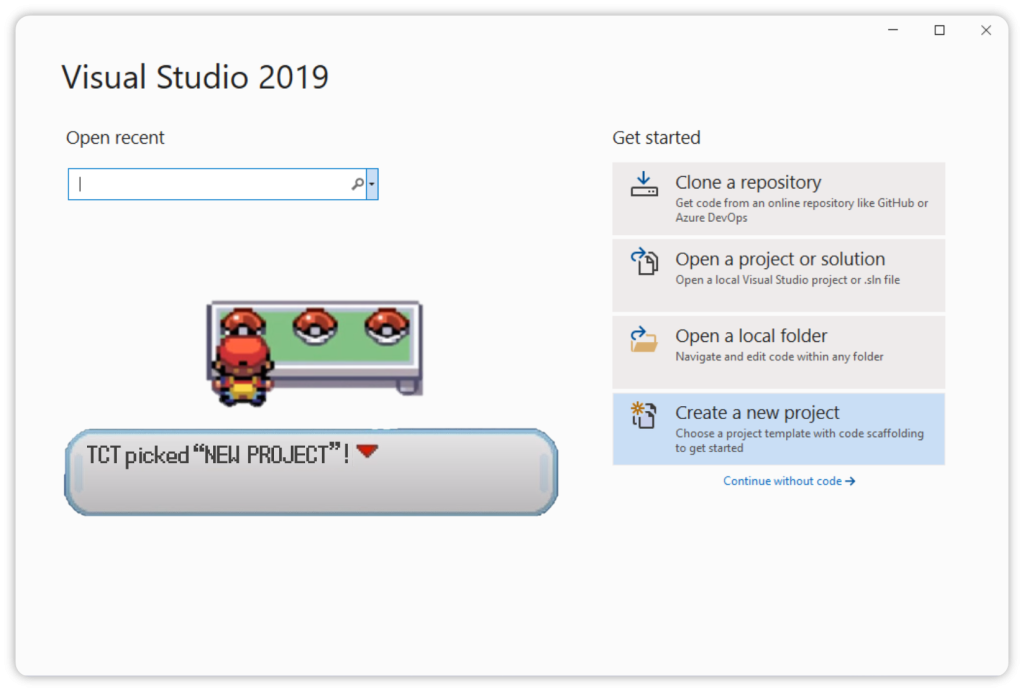
Next, select a Dot Net Standard Class Library with the C# icon present in it. Automation Anywhere doesn’t support VB.Net so skip that.
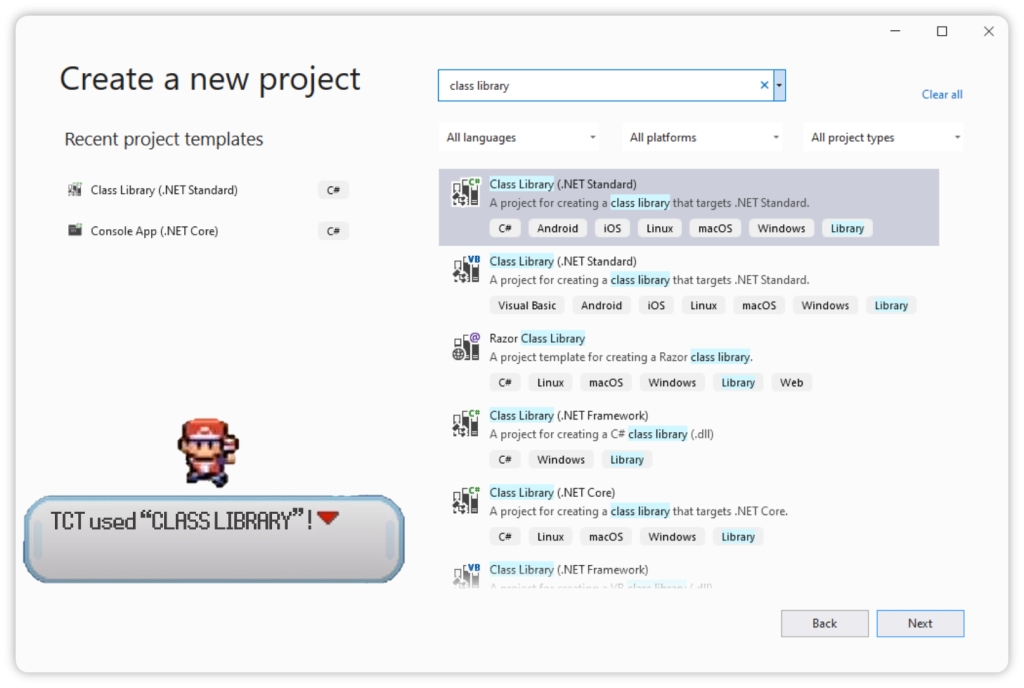
Now provide a suitable name.
This will appear as the Namespace.
Don’t think too much about it what all of this means – devote your attention to the process.
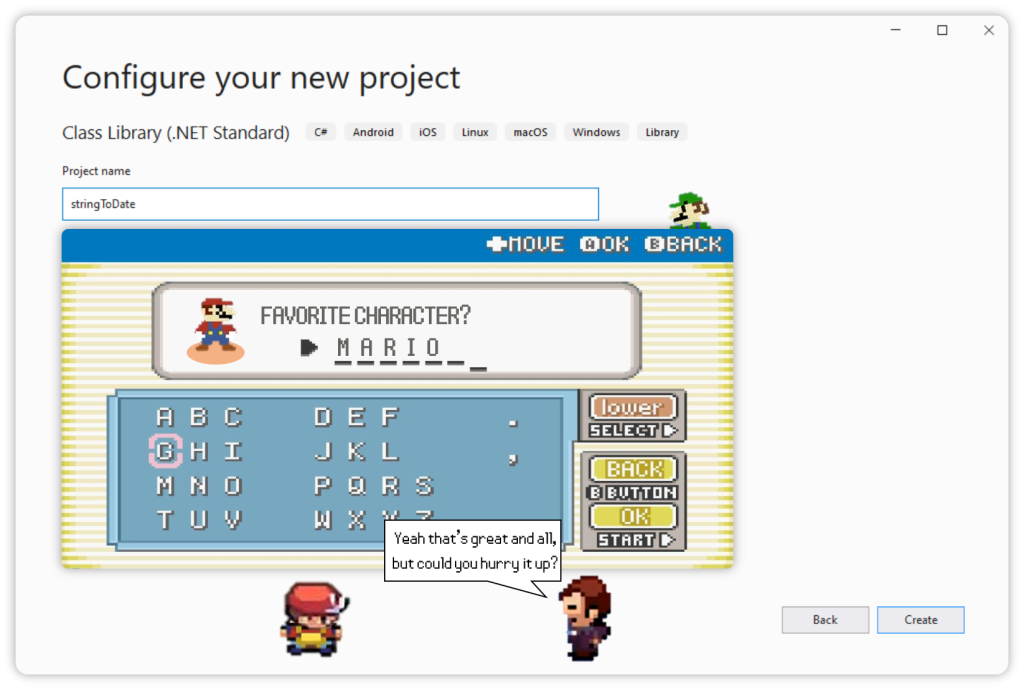
Even my characters have started going off topic.
Once its done loading, this is what you will see.
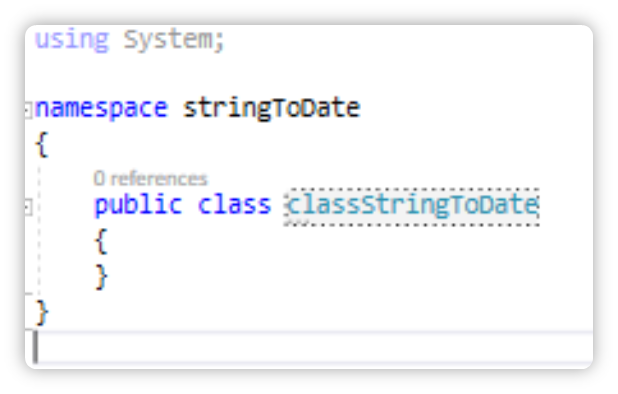
I went ahead and changed the Class name to classStringToDate just so that I can recognize it. You can give any name you like, but make sure you remember them.
Next, lets create our first Method!
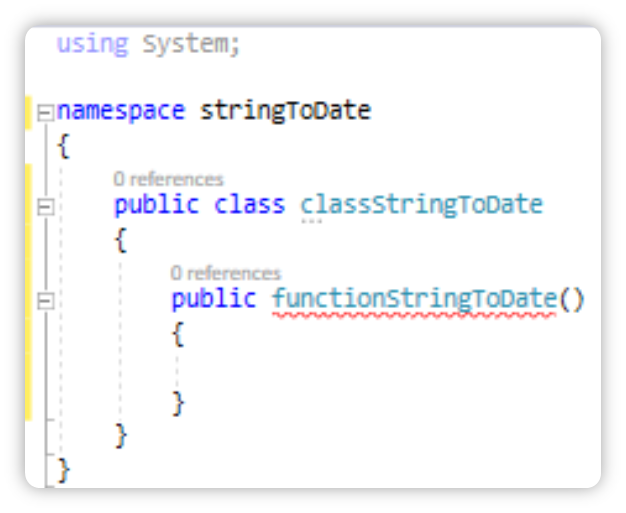
I have declared a Public Method called functionStringToDate.
The class will only cooperate with us, if we tell it to go public with its stocks.
Again, provide any name you like, since we are just exploring.
Before we proceed any further, let’s talk a little about the solution we are about to implement.
What exactly happens here?
It will receive a string and convert that to a desired date format and send it back, but how do we translate this into technical terms?
Is it possible for a method to return a variable of type DateTime in another format?
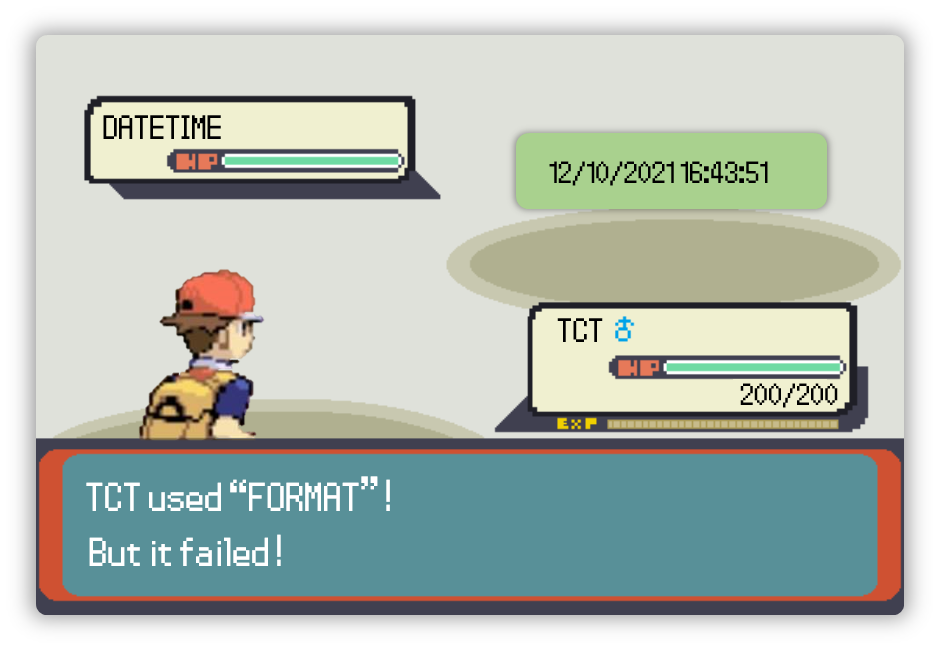
No and that is because the DateTime variable has a fixed format. You can only convert it to a desired format when that operation includes string conversion.
So, how do we go about achieving our solution?
For a start, we have to first convert that string into DateTime, before we can convert it into the format we want.
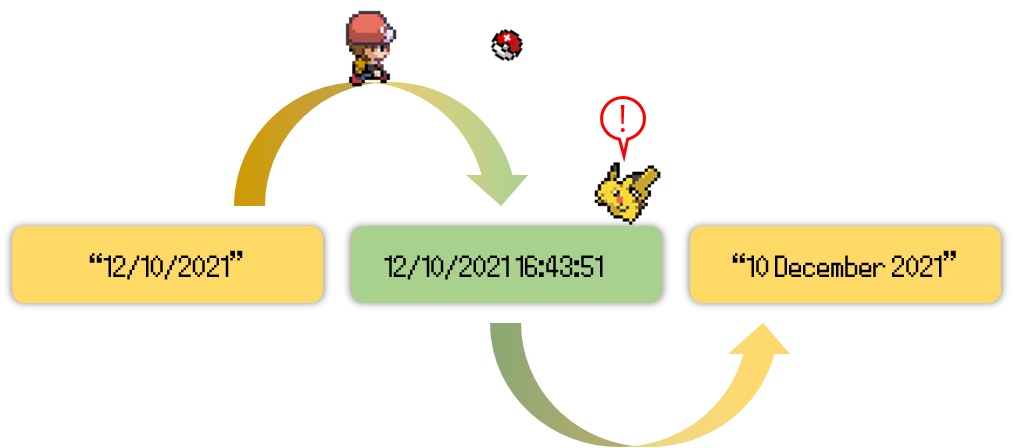
But before we perform any sort of conversion, we have to pass those items in as parameters.
We have to pass in a date which is in string format and also specify what format that particular string is in.
“12/10/2021” //format is MM/dd/yyyy
Oh but that’s not all.
We also have to pass in the “desired format” as well, which makes a total of three parameters.
public string functionStringToDate(string inputDate, string dateFormat, string desiredDateFormat)
We are making everything public, because if we don’t then we won’t be able to use them.
Now for the logic that will stitch our solution together.
Initializing Variables
We will be introducing few more members into the family.
When you initialize a variable, you are allocating memory to them. Without this, you won’t be able to use them.
DateTime dtCheck, dtConvert;
string res;
Some prefer initializing with var, but since I know what values I am going to put into them, it doesn’t really matter.
Also right now we have five variables to play around with. We just created two right now, while the other three are accepted into the method.
So let’s play around with them, now shall we?
Parsing Strings to Date
Although Convert.ToDateTime(“Date Goes Here”) works, there are instances where it doesn’t.
We will use the Parse Method to get what we want, and we will be using it Twice.
The first time, would be to check whether the entered String and date Format match, while the second time would be to perform the conversion and return it back to Automation Anywhere.
If either the string or format is wrong, we have to handle it accordingly.
This check will be encased inside of an If Block:
if (!DateTime.TryParseExact(inputDate, dateFormat, System.Globalization.CultureInfo.InvariantCulture, DateTimeStyles.None, out dtCheck))
{
res = "Invalid date/format was supplied, please check and try again.";
}
The TryParseExact method returns False if it fails to perform the conversion, which is why I have used it here.
If you have noticed, I have included an exclamation mark which tells the compiler that I want the block to get executed if the value returned is False.
System.Globalization.CultureInfo.InvariantCulture //The heck is this?
The third month of the year is called “March” in English, while it is called “Mars” in French. That is specified by the CultureInfo, but since we are dealing with English, we don’t have to worry about it. The CultureInfo is not important here which is why we have invoked CultureInvariant.
If you are interested in learning more, then head over to the link embedded in the image below.
Now Onto the Conversion
If the conversion succeeds, the condition will return true and it will exit the if block…unless we add an else.
if (!DateTime.TryParseExact(inputDate, dateFormat, System.Globalization.CultureInfo.InvariantCulture, DateTimeStyles.None, out dtCheck))
{
res = "Invalid date/format was supplied, please check and try again.";
}
else
{
//Logic for conversion goes here
}
The else block will contain a snippet that performs the conversion for us.
else
{
dtConvert = DateTime.ParseExact(inputDate, dateFormat, System.Globalization.CultureInfo.InvariantCulture);
res = dtConvert.ToString(desiredDateFormat);
}
The conversion is performed using a ParseExact method, and the format is applied during string conversion in the second line.
Is That All?
Although we have finished with the logic, we still have to encase it inside a try-catch.
The condition checking actually takes care of everything for us, but it’s always better to stay on the safer side by doing so.
This is what the entire code will look like:
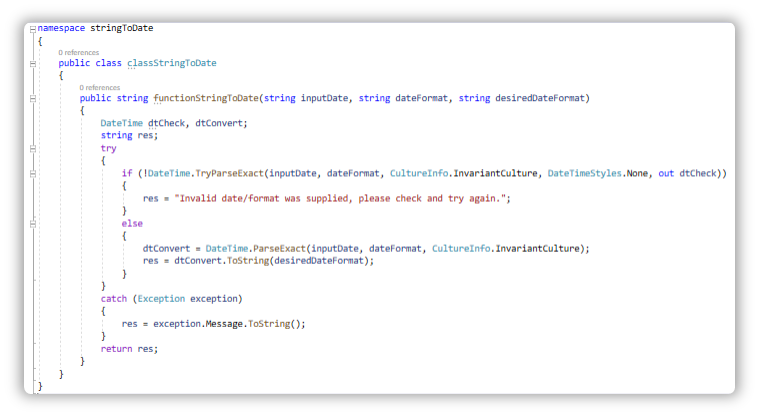
All thats left is to build the solution and locate the DLL.
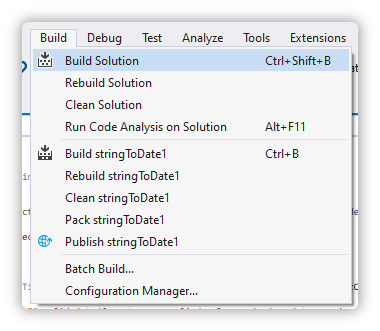
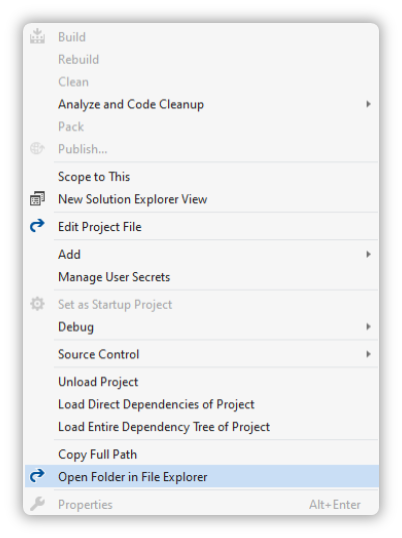
You will find the DLL nested in the path provided below:
C:\Users\AA\source\repos\stringToDate1\bin\Debug\netstandard2.0
And with that, you have developed your first C# program!
Back To the Control Room, Fellow Dabble-oper
Time to test out your Dabble-opment.
Ideally, it would be best to create a Console, add the DLL either as a reference, or into the same directory as the Console and test it out from there before trying it out in Automation Anywhere.
Lets head back to the Control Room.
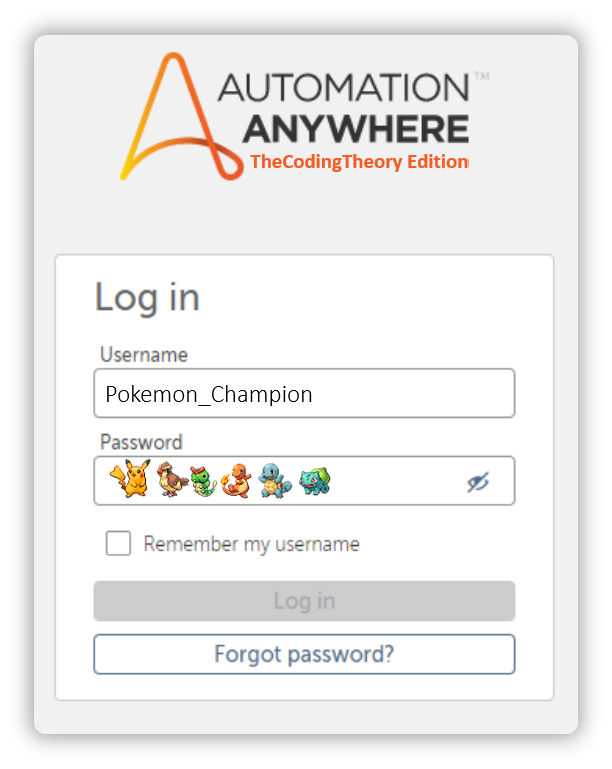
The DLL Actions use something called a Session Variable, which can either be local, global or variable. I went ahead and created a DLL Session Variable. This isn’t necessary, as you can simply pass a string into the Local Session section.
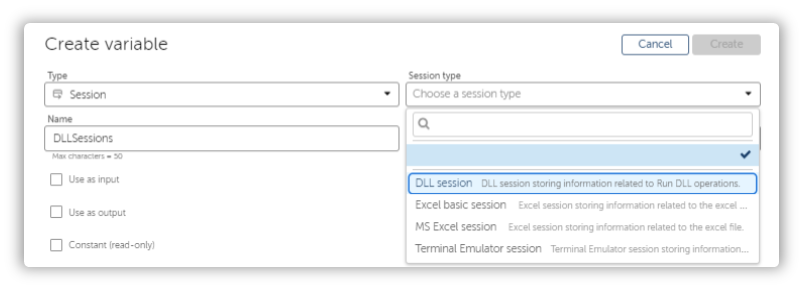
Now drag in Three DLL Action:
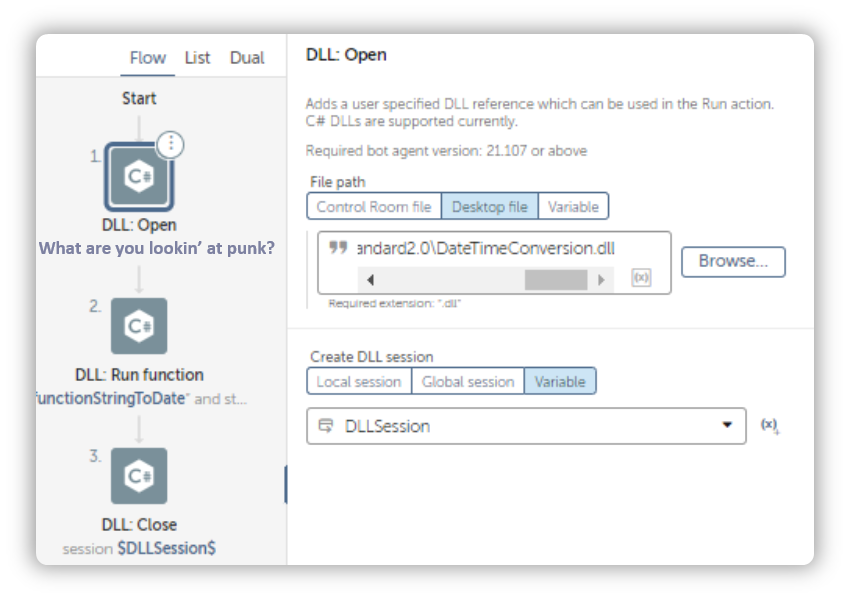
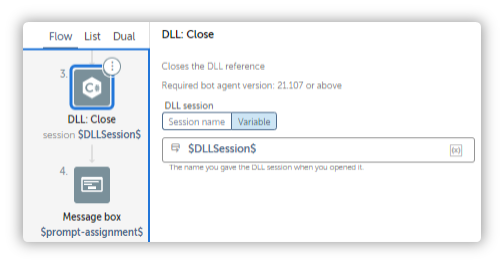
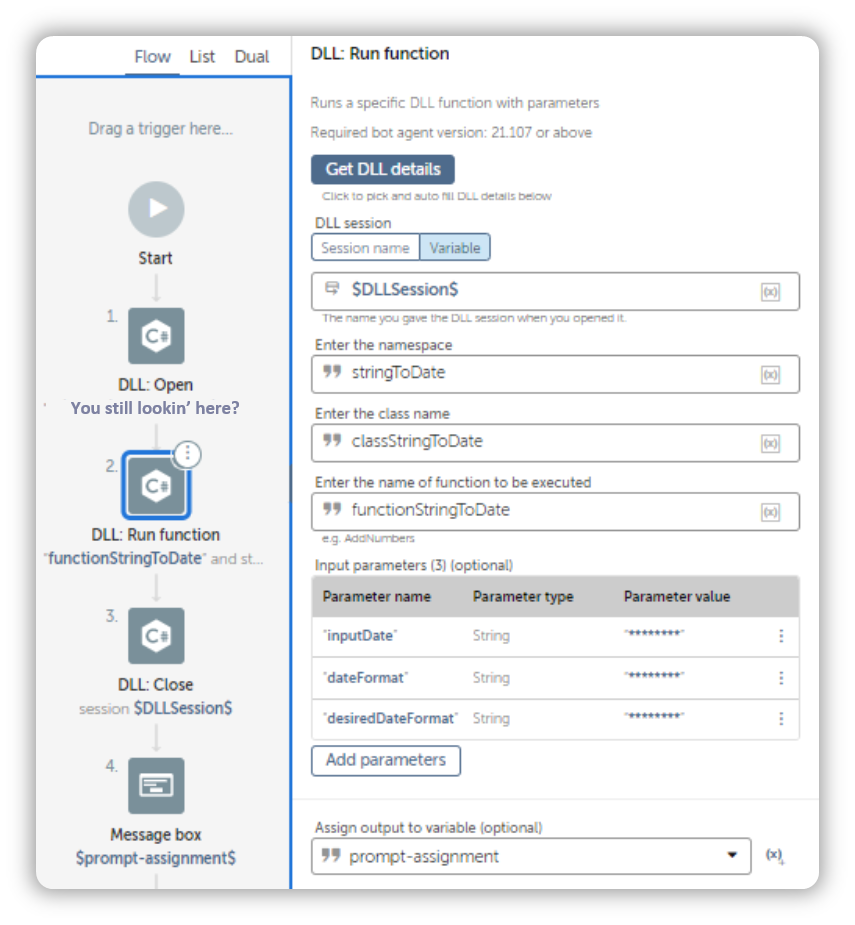
Zooming into the Run Action
The Run action is a little interesting.
The Get DLL Details button does most of the work for us.
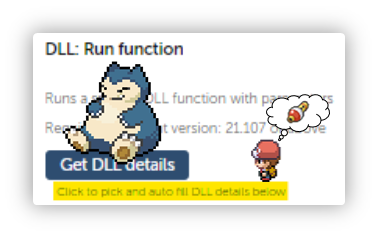
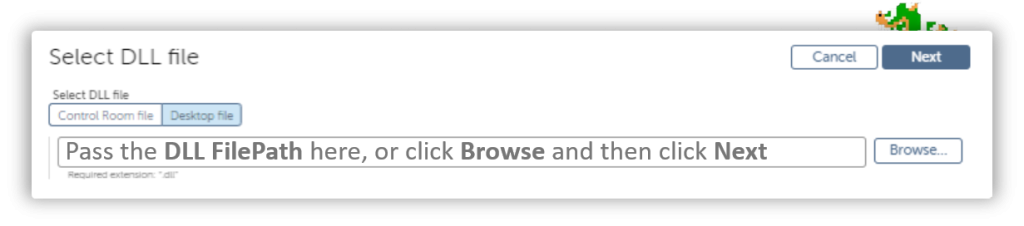
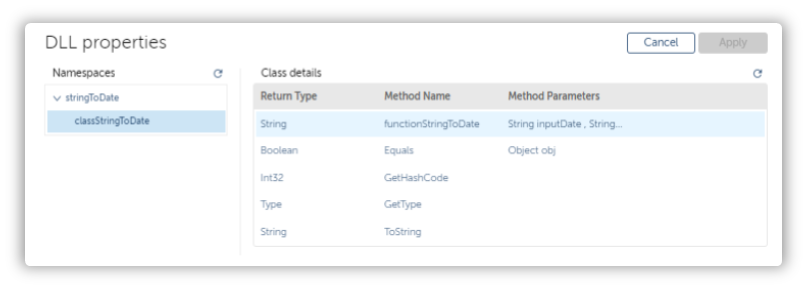
As you can see, most of the information was filled in by the Get DLL Details.
All that’s left for us is to pass in the parameters.
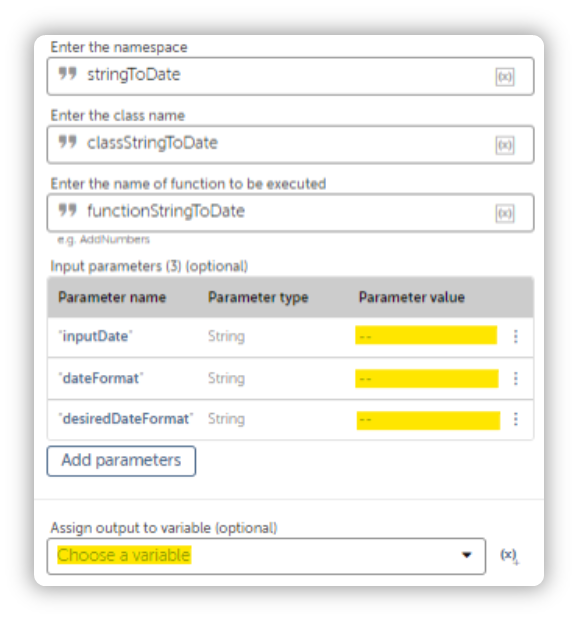
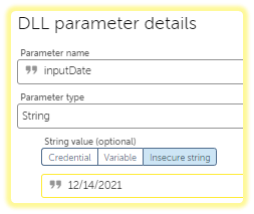
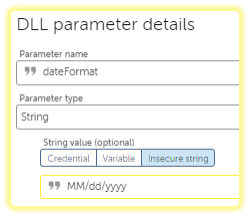
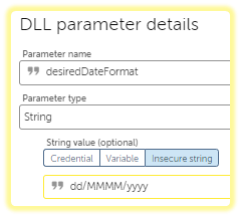
Let’s run our automation and see what turns up.
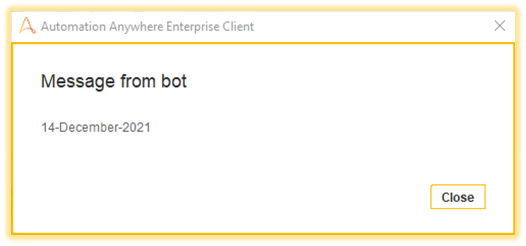
How about we feed an incorrect format? Is our dabble-opment capable enough to handle exceptions?
Lets find out!
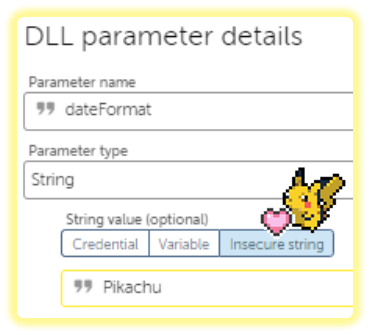
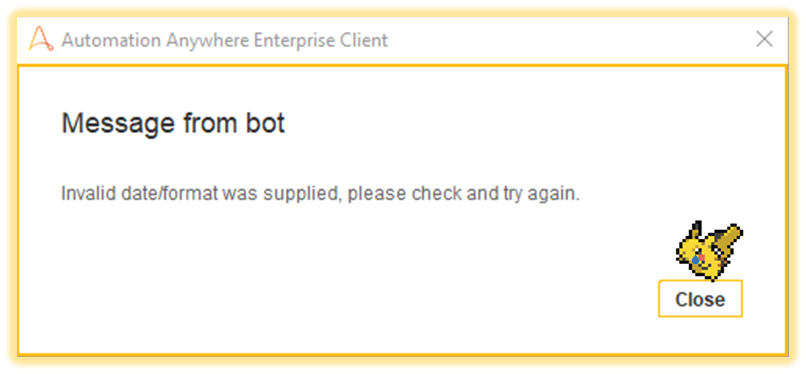
Atleast we handled it…right?
And thats pretty much it.
I hope you’ve learnt something from this.
I will explore more exercises using DLLs in the coming days so as always,
Stay tuned.
Pretty humorous clear steps… All ready to dabble-dabble DLLs with AA!!
Hi Ashwini,
Appreciate the feedback!
Kind Regards,
TCT